Физические безумства01.05.2019 22:47
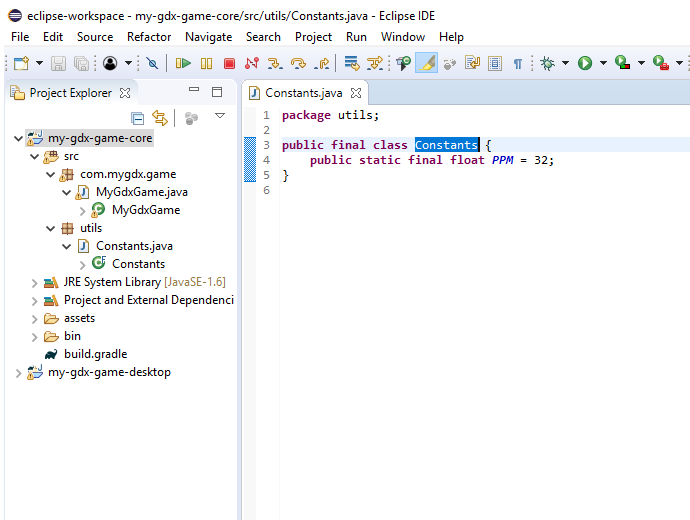
package com.mygdx.game;
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.physics.box2d.FixtureDef;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.physics.box2d.Body;
import com.badlogic.gdx.physics.box2d.BodyDef;
import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer;
import com.badlogic.gdx.physics.box2d.CircleShape;
import com.badlogic.gdx.physics.box2d.PolygonShape;
import com.badlogic.gdx.physics.box2d.World;
import utils.Constants;
public class MyGdxGame extends ApplicationAdapter {
SpriteBatch batch;
Texture img;
private OrthographicCamera camera;
private boolean DEBUG = false;
private World world;
private Body ball;
private Body floor;
private Body wall;
private Body verticals;
private Body horizontals;
private Box2DDebugRenderer b2dr;
public void create() {
float w = Gdx.graphics.getWidth();
float h = Gdx.graphics.getHeight();
camera = new OrthographicCamera();
camera.setToOrtho(false, w / 2, h / 2);
world = new World(new Vector2(0, -9.8f), false);
b2dr = new Box2DDebugRenderer();
// наш мячик
ball = createPlayer();
// границы мира
floor = createfloor();
wall = createwall(100, 170);
wall = createwall(-60, 170);
// создаем мячики снизу. Через цикл пробовал, не работает
verticals = createverticals(5, 50);
verticals = createverticals(10, 50);
verticals = createverticals(15, 50);
verticals = createverticals(20, 50);
verticals = createverticals(25, 50);
verticals = createverticals(30, 50);
verticals = createverticals(35, 50);
verticals = createverticals(5, 55);
verticals = createverticals(10, 55);
verticals = createverticals(15, 55);
verticals = createverticals(20, 55);
verticals = createverticals(25, 55);
verticals = createverticals(30, 55);
verticals = createverticals(35, 55);
verticals = createverticals(5, 60);
verticals = createverticals(10, 60);
verticals = createverticals(15, 60);
verticals = createverticals(20, 60);
verticals = createverticals(25, 60);
verticals = createverticals(30, 60);
verticals = createverticals(35, 60);
verticals = createverticals(5, 70);
verticals = createverticals(10, 70);
verticals = createverticals(15, 70);
verticals = createverticals(20, 70);
verticals = createverticals(25, 70);
verticals = createverticals(30, 70);
verticals = createverticals(35, 70);
verticals = createverticals(5, 80);
verticals = createverticals(10, 80);
verticals = createverticals(15, 80);
verticals = createverticals(20, 80);
verticals = createverticals(25, 80);
verticals = createverticals(30, 80);
verticals = createverticals(35, 80);
}
public void render() {
update(Gdx.graphics.getDeltaTime());
Gdx.gl.glClearColor(0, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
b2dr.render(world, camera.combined.scl(Constants.PPM));
}
public void resize(int width, int height) {
camera.setToOrtho(false, width / 2, height / 2);
}
public void dispose() {
world.dispose();
b2dr.dispose();
}
public void update(float delta) {
world.step(1 / 60f, 6, 2);
inputUpdate(delta);
cameraUpdate(delta);
}
// чтобы мяч вращался
public void inputUpdate(float delta) {
ball.setAngularVelocity(3.0f);
}
public void cameraUpdate(float delta) {
Vector3 position = camera.position;
position.x = ball.getPosition().x * Constants.PPM;
position.y = ball.getPosition().y * Constants.PPM;
camera.position.set(position);
camera.update();
}
// создаем мячик
public Body createPlayer() {
Body pBody;
BodyDef def = new BodyDef();
def.type = BodyDef.BodyType.DynamicBody;
def.position.set(20 / Constants.PPM, 800 / Constants.PPM);
def.fixedRotation = false;
pBody = world.createBody(def);
CircleShape shape = new CircleShape();
shape.setRadius(10 / Constants.PPM);
pBody.createFixture(shape, 1.0f);
def.bullet = true;
shape.dispose();
return pBody;
}
// создаем пол
public Body createfloor() {
Body fBody;
BodyDef def = new BodyDef();
def.type = BodyDef.BodyType.StaticBody;
def.position.set(0, 0);
def.fixedRotation = true;
fBody = world.createBody(def);
PolygonShape shape = new PolygonShape();
shape.setAsBox(480 / Constants.PPM, 70 / Constants.PPM);
fBody.createFixture(shape, 0.001f);
shape.dispose();
return fBody;
}
// создаем стены
public Body createwall(int xo, int yo) {
Body fBody;
BodyDef def = new BodyDef();
def.type = BodyDef.BodyType.StaticBody;
def.position.set(xo / Constants.PPM, yo / Constants.PPM);
def.fixedRotation = true;
fBody = world.createBody(def);
PolygonShape shape = new PolygonShape();
shape.setAsBox(50 / Constants.PPM, 100 / Constants.PPM);
fBody.createFixture(shape, 0.001f);
shape.dispose();
return fBody;
}
// обратите здесь внимание на параметры
public Body createverticals(int xo, int yo) {
Body pBody;
BodyDef def = new BodyDef();
def.type = BodyDef.BodyType.DynamicBody;
def.position.set(xo / Constants.PPM, yo / Constants.PPM);
def.fixedRotation = false;
pBody = world.createBody(def);
CircleShape shape = new CircleShape();
shape.setRadius(2 / Constants.PPM);
// здесь заключена вся магия
FixtureDef fd = new FixtureDef();
// упругость
fd.restitution = 1.0f;
// плотность
fd.density = 5.0f;
// трение
fd.friction = 0.01f;
fd.shape = shape;
pBody.createFixture(fd);
def.bullet = true;
shape.dispose();
return pBody;
}
}
© Habrahabr.ru