Как построить мастабу01.04.2024 00:45
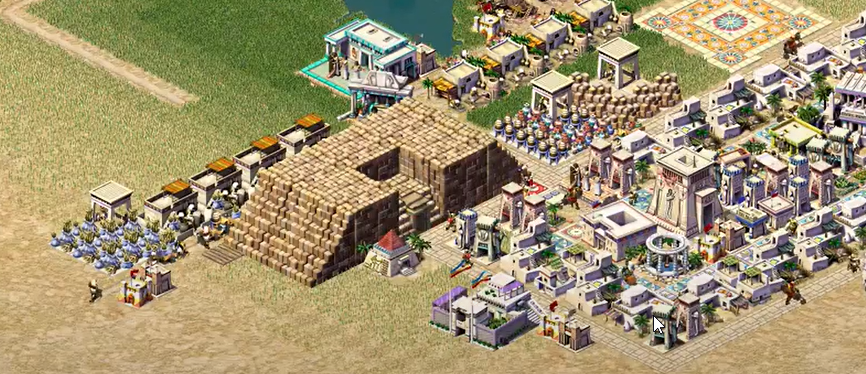
void draw_mastaba_base_tiles(int type, int x, int y, int orientation) {
int flooring_image_id = image_id_from_group(GROUP_BUILDING_MASTABA_FLOORING, type);
int side1_image_id = image_id_from_group(GROUP_BUILDING_MASTABA_side_1, type);
int side2_image_id = image_id_from_group(GROUP_BUILDING_MASTABA_side_2, type);
int EMPTY = 0;
// floor tiles
int til_0 = flooring_image_id + 0;
int til_1 = flooring_image_id + 1;
int til_2 = flooring_image_id + 2;
int til_3 = flooring_image_id + 3;
// small (1x1) sides
int smst0 = side1_image_id + (4 - city_view_orientation() / 2) % 4; // north
int smst1 = side1_image_id + (5 - city_view_orientation() / 2) % 4; // east
int smst2 = side1_image_id + (6 - city_view_orientation() / 2) % 4; // south
int smst3 = side1_image_id + (7 - city_view_orientation() / 2) % 4; // west
// long (1x2) sides
int lst0B = side2_image_id + (8 - city_view_orientation()) % 8; // north
int lst0A = side2_image_id + (9 - city_view_orientation()) % 8;
int lst1B = side2_image_id + (10 - city_view_orientation()) % 8; // east
int lst1A = side2_image_id + (11 - city_view_orientation()) % 8;
int lst2B = side2_image_id + (12 - city_view_orientation()) % 8; // south
int lst2A = side2_image_id + (13 - city_view_orientation()) % 8;
int lst3B = side2_image_id + (14 - city_view_orientation()) % 8; // west
int lst3A = side2_image_id + (15 - city_view_orientation()) % 8;
// correct long sides graphics for relative orientation
switch (city_view_orientation() / 2) {
case 1:
case 0:
lst1A = side2_image_id + (10 - city_view_orientation()) % 8; // east
lst1B = side2_image_id + (11 - city_view_orientation()) % 8;
lst3A = side2_image_id + (14 - city_view_orientation()) % 8; // west
lst3B = side2_image_id + (15 - city_view_orientation()) % 8;
break;
}
switch (city_view_orientation() / 2) {
case 3:
case 0:
lst0A = side2_image_id + (8 - city_view_orientation()) % 8; // north
lst0B = side2_image_id + (9 - city_view_orientation()) % 8;
lst2A = side2_image_id + (12 - city_view_orientation()) % 8; // south
lst2B = side2_image_id + (13 - city_view_orientation()) % 8;
break;
}
// adjust northern tile offset
tile2i north_tile = {x, y};
switch (orientation) {
case 0: // NE
north_tile.shift(-2, -10);
// north_tile.x -= 2;
// north_tile.y -= 10;
break;
case 1: // SE
north_tile.shift(0, -2);
// north_tile.y -= 2;
break;
case 2: // SW
north_tile.shift(-2, 0);
// north_tile.x -= 2;
break;
case 3: // NW
north_tile.shift(-10, -2);
// north_tile.x -= 10;
// north_tile.y -= 2;
break;
}
// first, add base tiles
switch (orientation) {
case 0: { // NE
int MASTABA_SCHEME[13][5] = {
{til_3, lst1A, lst1B, til_1, lst3A},
{til_2, lst1A, lst1B, til_1, lst3A},
{til_3, lst1A, lst1B, til_1, lst3A},
{til_2, til_0, til_0, til_1, til_0},
{til_0, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{til_1, til_1, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{til_1, til_1, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
};
for (int row = 0; row < 13; row++) {
for (int column = 0; column < 5; column++)
map_image_set(MAP_OFFSET(north_tile.x() + column, north_tile.y() + row),
MASTABA_SCHEME[row][column]);
}
break;
}
case 1: { // SE
int MASTABA_SCHEME[5][13] = {
{smst0, smst0, til_1, smst0, smst0, til_1, smst0, smst0, til_0, til_2, til_3, til_2, til_3},
{til_0, til_0, til_1, til_0, til_0, til_1, til_0, til_0, til_0, til_0, lst2B, lst2B, lst2B},
{EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, til_0, lst2A, lst2A, lst2A},
{EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, til_1, til_1, til_1, til_1},
{EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, til_0, lst0B, lst0B, lst0B},
};
for (int row = 0; row < 5; row++) {
for (int column = 0; column < 13; column++)
map_image_set(MAP_OFFSET(north_tile.x() + column, north_tile.y() + row),
MASTABA_SCHEME[row][column]);
}
break;
}
case 2: { // SW
int MASTABA_SCHEME[13][5] = {
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{til_1, til_1, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{til_1, til_1, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{smst3, til_0, EMPTY, EMPTY, EMPTY},
{til_0, til_0, EMPTY, EMPTY, EMPTY},
{til_2, til_0, til_0, til_1, til_0},
{til_3, lst1A, lst1B, til_1, lst3A},
{til_2, lst1A, lst1B, til_1, lst3A},
{til_3, lst1A, lst1B, til_1, lst3A},
};
for (int row = 0; row < 13; row++) {
for (int column = 0; column < 5; column++)
map_image_set(MAP_OFFSET(north_tile.x() + column, north_tile.y() + row),
MASTABA_SCHEME[row][column]);
}
break;
}
case 3: { // NW
int MASTABA_SCHEME[5][13] = {
{til_3, til_2, til_3, til_2, til_0, smst0, smst0, til_1, smst0, smst0, til_1, smst0, smst0},
{lst2B, lst2B, lst2B, til_0, til_0, til_0, til_0, til_1, til_0, til_0, til_1, til_0, til_0},
{lst2A, lst2A, lst2A, til_0, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY},
{til_1, til_1, til_1, til_1, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY},
{lst0B, lst0B, lst0B, til_0, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY},
};
for (int row = 0; row < 5; row++) {
for (int column = 0; column < 13; column++)
map_image_set(MAP_OFFSET(north_tile.x() + column, north_tile.y() + row),
MASTABA_SCHEME[row][column]);
}
break;
}
}
}
© Habrahabr.ru