How to do exponents in Java
Java doesn«t have an exponents operator like »**» in Python, but you can still calculate exponents in a different way. You can make exponents in Java with Math.pow (), it«s a good option for floating point numbers, or you can use BigInteger.pow () for large ones.
You can also use for-loop and while-loop to do exponents, but the Math.pow () is the most suitable, in my opinion, as it can be used to measure power for any number, both negative and positive. Moreover, it doesn«t require you to define user-defined logic, which means you don«t need to write your own loop or define a recursive function. That saves your time while coding and makes the code easier to read.
Different ways to do exponents in Java
- Math.pow (): It«s part of Java«s built-in Math class. Easy to use, handles both integer and floating-point numbers. And can handle both positive and negative exponents. However, it returns in double so that they may be errors in extremely large fractions/numbers.
BigInteger.pow (): It«s part of Java«s BigInteger class. It works only if you must deal with large values and accept only positive integer exponents. It«s also slower and uses much more memory compared to Math class. For-loop: That«s a manual method, so I don«t recommend it. It works only with non-negative number exponents. It also doesn«t require additional classes, but in my opinion, is less efficient compared to Math.pow (). We can define arguments as int, so it would work with integers only, or as double, so it will work with both integers and floating-point numbers. While-loop: Almost the same as for-loop, but uses, obviously, while-loop instead. The same as for-loop, it works only with non-negative numbers.
Method | Floating-point numbers | Negative exponents | Very large numbers | Efficiency |
---|---|---|---|---|
Math.pow () | Yes | Yes | No | High |
BigInteger.pow () | No | No | Yes | Low |
For-loop | No | No | Depends on the JVM | Medium |
While-loop | No | No | Depends on the JVM | Medium |
How to do exponents with Math.pow ()
The Math class is part of the java.lang package is pre-built; it«s implicitly imported, java.lang. Java automatically imports classes. So, you don«t need to write import java.lang.Math; and you can just use Math.pow () and any other methods from Math class.
The syntax of Math.pow () is simple; it includes two arguments: the base and the exponent. The base is the number you«re rising to power; the exponent is the power to raise the number.
Here«s how it works:
double base = 2.0;
double exponent = 3.0;
double result = Math.pow(base, exponent);
System.out.println("The exponent is: " + result);
Here:
- Math is the class name containing the pow () method.
- pow () is the method to perform exponentiation. It«s static, and you call the Math class itself without the need to create a class instance.
base and exponent are two parameters; the first is the number to raise to power; the second is the power itself. I used 2 as the base and 3 as the power. The mathematical equation would be x=2^3 Math.pow (base, exponent) executes the raise of the base into power (exponent) and assigns the result to the result variable.
If you try this code in the compiler, it may not work, giving you the output that: «At least one public class is required in the main file.»
In this case, the right structure for the code would be:
public class Main {
public static void main(String[] args) {
double base = 2.0;
double exponent = 3.0;
double result = Math.pow(base, exponent);
System.out.println("The exponent is: " + result);
}
}
Here:
- public means the method is accessible anywhere, including from outside the class it«s declared in. That«s necessary for JVM (Java Virtual Machine) to call this method.
- class declares the class in Java.
- Main is the class name.
{ is the opening that marks the beginning of the class definition; everything between the opening and closing brace would be part of the class. static means that the method belongs to the class itself. So the JVM would call the class itself. void means that the method isn«t returning anything back. main is the name of the method. String[] args) are parameters of the method. Here we have one parameter in an array of Strings. Now you can provide command-line arguments, and these arguments are passed to your main method through this parameter.
Here«s the code executed:
How to do exponents with BigInteger.pow ()
BigInteger.pow () is a method in the BigInteger class in Java, and it can be used to do exponents on very large values.
The BigInteger class belongs to java.math package.
The pow () method to do exponents has the following syntax:
public BigInteger pow(int exponent)
Here«s full code to execute:
import java.math.BigInteger;
public class Main {
public static void main(String[] args) {
BigInteger base = new BigInteger("20");
BigInteger result = base.pow(10);
System.out.println("The result is: " + result);
}
}
- import java.math.BigInteger; states that Java needs to include BigInteger class from the java.math package into the current file.
After you import BigInteger from java.math package, you can use BigInteger directly in your code without referring to java.math.BigInteger
- BigInteger is the class that contains the pow () method.
- You call the pow () method on an instance of the BigInteger class.
- BigInteger instances are immutable, so they can«t be altered once the instance is created.
pow () is the method to perform the exponentiation. exponent is the integer argument. That«s our exponent to which the base would be raised. It should be non-negative. BigInteger base = new BigInteger («base»); creates a new instance for BigInteger class that represents the integer. BigInteger result = base.pow (power); calls a pow () method on the base to raise it to power. The result would be stored as a result variable.
Here«s the code for BigInteger.pow () executed:
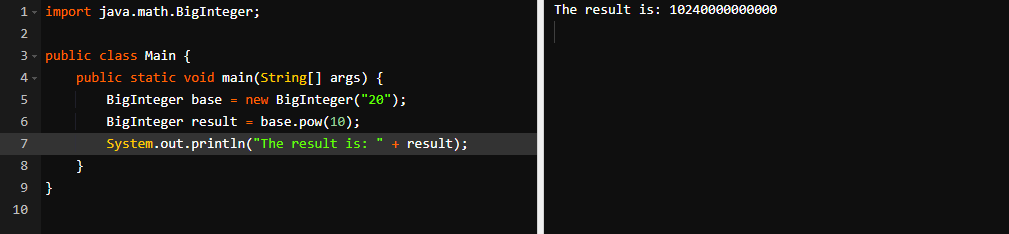
How to do an exponent with for-loop
The for-loop isn«t the best one to use, but it may be suitable if you know the number of iterations.
In the case of for-loop operation, the power should be greater than 1.
Here«s an example of code for base 2, power 3:
public class Main {
public static void main(String[] args) {
int base = 2;
int exponent = 3;
int result = 1;
for (int i = 0; i < exponent; i++) {
result = result * base;
}
System.out.println("The exponent is: " + result);
}
}
Here«s the code executed:
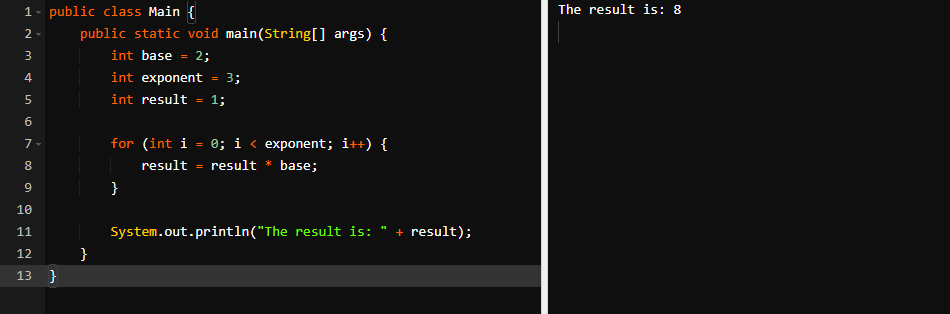
This code uses int for base and exponent, assuming that the number should be an integer. But you can also use double, to allow floating-point numbers. But you can define a double only for a base; that won«t work for an exponent; you still need to convert it to int, and if even we define it as double and then convert it to int, this will truncate any decimal portion of the exponent.
public class Main {
public static void main(String[] args) {
double base = 2.5;
int exponent = 3;
double result = 1.0;
for (int i = 0; i < exponent; i++) {
result *= base;
}
System.out.println("The exponent is: " + result);
}
}
- public class Main {
Here we declare a public class named Main.
- public static void main (String[] args) {
Here we declare the start of JVM execution; the main method is declared as public (meaning the method is accessible anywhere), static (the method belongs to the class itself), and void (the method isn«t returning anything back).
- double declares the base variable as a type of floating-point number that can hold decimal values.
- exponent is declared as an int so that it can be only an integer.
- result is declared as double.
for (int i = 0; i < exponent; i++) {
This line starts for-loop, which would be run from i=0 to i=exponent-1, as the condition is i < exponent; for each iteration, it would be incremented by 1 (i++)
- result *= base;
In each iteration of the loop, the result value would be multiplied by the base. The result would then be assigned back to the result. Like result = result*base.
In our case, it«s 3*(2.5)=2.5×2.5×2.5
- System.out.println («The exponent is:» + result);
Displays the final number of the result to the console.
The code executed:
How to do an exponent with while-loop
A for-loop and a while-loop in Java are used to perform almost the same task — to execute a code. But there«s one key difference: a loop variable is declared and initialized directly in the declaration, and the condition is checked in the beginning. That«s great when you know the exact number of loop rounds beforehand.
In a while loop, you need to declare and initialize the variable before the loop itself, and the condition is checked before each iteration. That can be used when you don«t know the number of iterations beforehand, so the loop needs to proceed until the condition is met.
Here«s is an example of code to do an exponent with a while-loop; the base is declared as double so that it can be a floating-point number:
public class Main {
public static void main(String[] args) {
double base = 2.5;
int exponent = 3;
double result = 1.0;
while (exponent > 0) {
result *= base;
exponent--;
}
System.out.println("The exponent is: " + result);
}
}
From a mathematical point of view, it looks like this:
- We start with a base of 2.5, an exponent of 3, and a result of 1.0.
- We then enter the while loop. The condition for the while loop is exponent > 0. The exponent is 3 at the start, so we enter the loop.
- Inside the loop, we first perform the operation result *= base; This multiplies the result by the base and assigns the result back to the result. The base is 2.5, and the result is 1.0, so the result becomes 2.5×1.0 = 2.5.
We then decrement the exponent by 1 using the exponent– operation. The exponent is now 2. The program then goes back to the top of the while loop and rechecks the condition. Since the exponent is still greater than 0, we go through the loop again. The result is now 2.5×2.5 = 6.25, and the exponent is decremented to 1. We go through the loop one more time. The result is 6.25×2.5 = 15.625, and the exponent is decremented to 0. We then return to the top of the while loop again. The exponent is not greater than 0 this time, so we do not enter the loop again. The final value of the result is 15.625, which is equal to 2.5 to the power of 3.
The code executed:
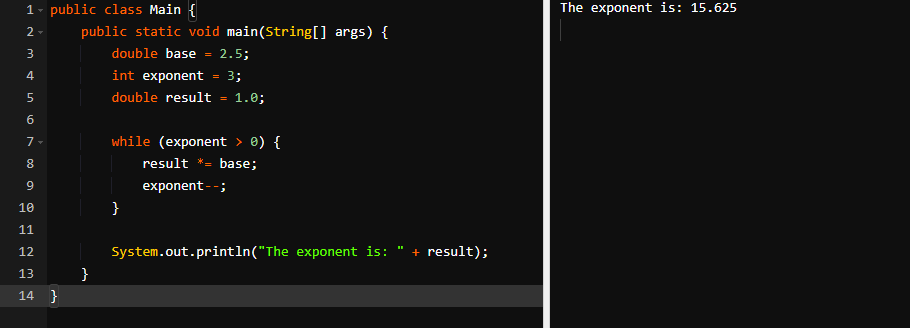
© tab-tv